step 1. Create a new website ...
new default page..
step 2.Go to solution explorer add new item. Add class and give name DAL it will ask for create new app_code click on yes it will automatic add a new folder.
It will look like this after click on yes
Step :3 Repeat Step 2 And Give the Name BAL.
Step:4.Design Your Page
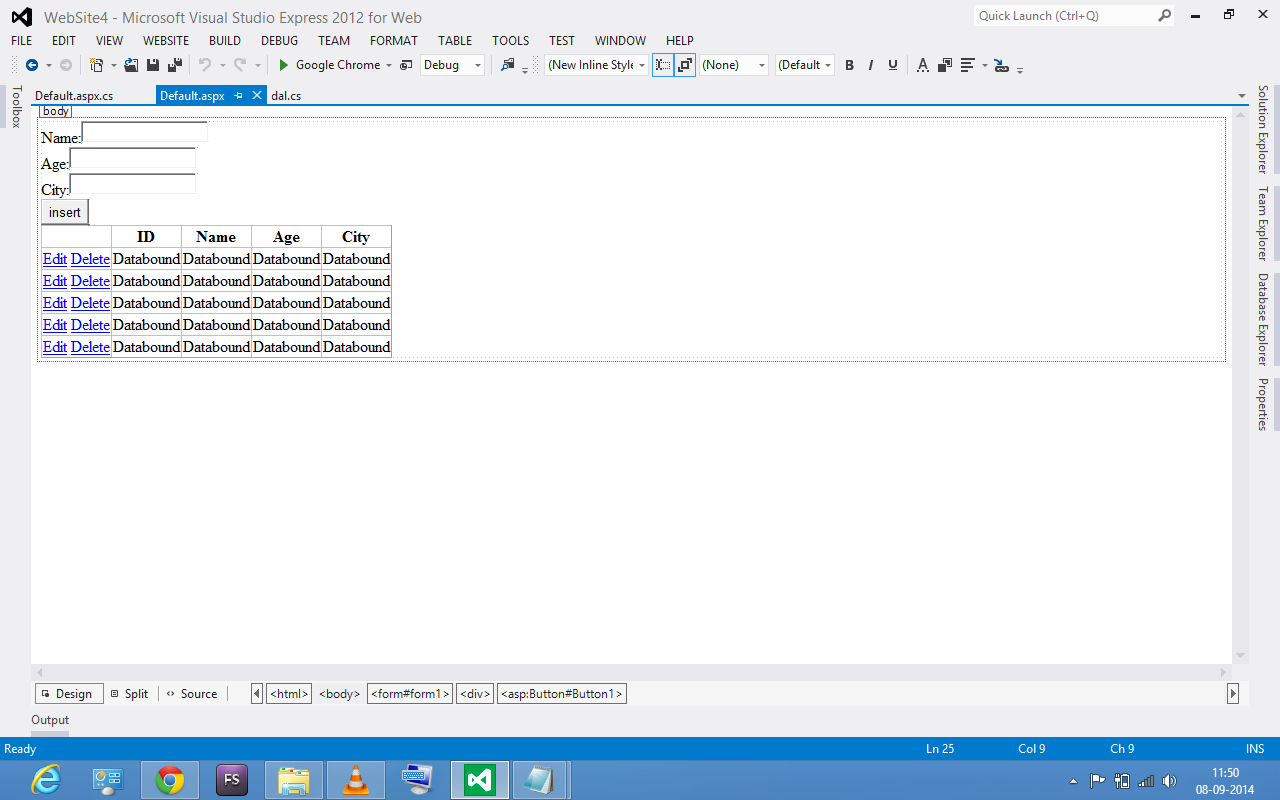
Step 5: In DAL File
Write that code You can difine your all logic here and also connection string
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
/// <summary>
/// Summary description for dal
/// </summary>
public class dal
{
SqlCommand cmd;
SqlConnection con;
SqlDataAdapter da;
DataSet ds;
public dal()
{
con = new SqlConnection(@"Data Source=TOPS-222\SQLEXPRESS;Initial Catalog=mytestdb;Integrated Security=True");
}
public DataSet disp()
{
cmd = new SqlCommand("disp",con);
cmd.CommandType = CommandType.StoredProcedure;
da = new SqlDataAdapter(cmd);
ds = new DataSet();
da.Fill(ds);
return ds;
}
public void insert(bal bl)
{
cmd = new SqlCommand("inserts", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("name",bl.name);
cmd.Parameters.AddWithValue("age", bl.age);
cmd.Parameters.AddWithValue("city", bl.city);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
public void update(bal bl)
{
cmd = new SqlCommand("updates", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("id",bl.id);
cmd.Parameters.AddWithValue("name", bl.name);
cmd.Parameters.AddWithValue("age", bl.age);
cmd.Parameters.AddWithValue("city", bl.city);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
public void delete(int a)
{
cmd = new SqlCommand("deletes", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("id", a);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
step 6:-In BAL File Create property and the create the DAL Object here.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
/// <summary>
/// Summary description for bal
/// </summary>
public class bal
{
public int id { get; set; }
public string name { get; set; }
public int age { get; set; }
public string city { get; set; }
dal dl = new dal();
public DataSet disp1()
{
return dl.disp();
}
public void insert1(bal bl)
{
dl.insert(bl);
}
public void update1(bal bl)
{
dl.update(bl);
}
public void delete1(int a)
{
dl.delete(a);
}
}
Step:7
.Default.aspx.cs Code File Write That Code..
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
bal bl = new bal();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
disp();
}
}
public void disp()
{
GridView1.DataSource = bl.disp1();
GridView1.DataBind();
}
protected void Button1_Click(object sender, EventArgs e)
{
bl.name = TextBox1.Text;
bl.age = int.Parse(TextBox2.Text);
bl.city = TextBox3.Text;
bl.insert1(bl);
disp();
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
int a = int.Parse(((Label)GridView1.Rows[e.RowIndex].FindControl("Label1")).Text);
bl.delete1(a);
disp();
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
disp();
}
protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex = -1;
disp();
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
bl.id = int.Parse(((Label)GridView1.Rows[e.RowIndex].FindControl("Label1")).Text);
bl.name = ((TextBox)GridView1.Rows[e.RowIndex].FindControl("TextBox2")).Text;
bl.age = int.Parse(((TextBox)GridView1.Rows[e.RowIndex].FindControl("TextBox3")).Text);
bl.city = ((TextBox)GridView1.Rows[e.RowIndex].FindControl("TextBox4")).Text;
bl.update1(bl);
GridView1.EditIndex = -1;
disp();
}
}
...
If you have any query then contact me
new default page..
step 2.Go to solution explorer add new item. Add class and give name DAL it will ask for create new app_code click on yes it will automatic add a new folder.
Step:4.Design Your Page
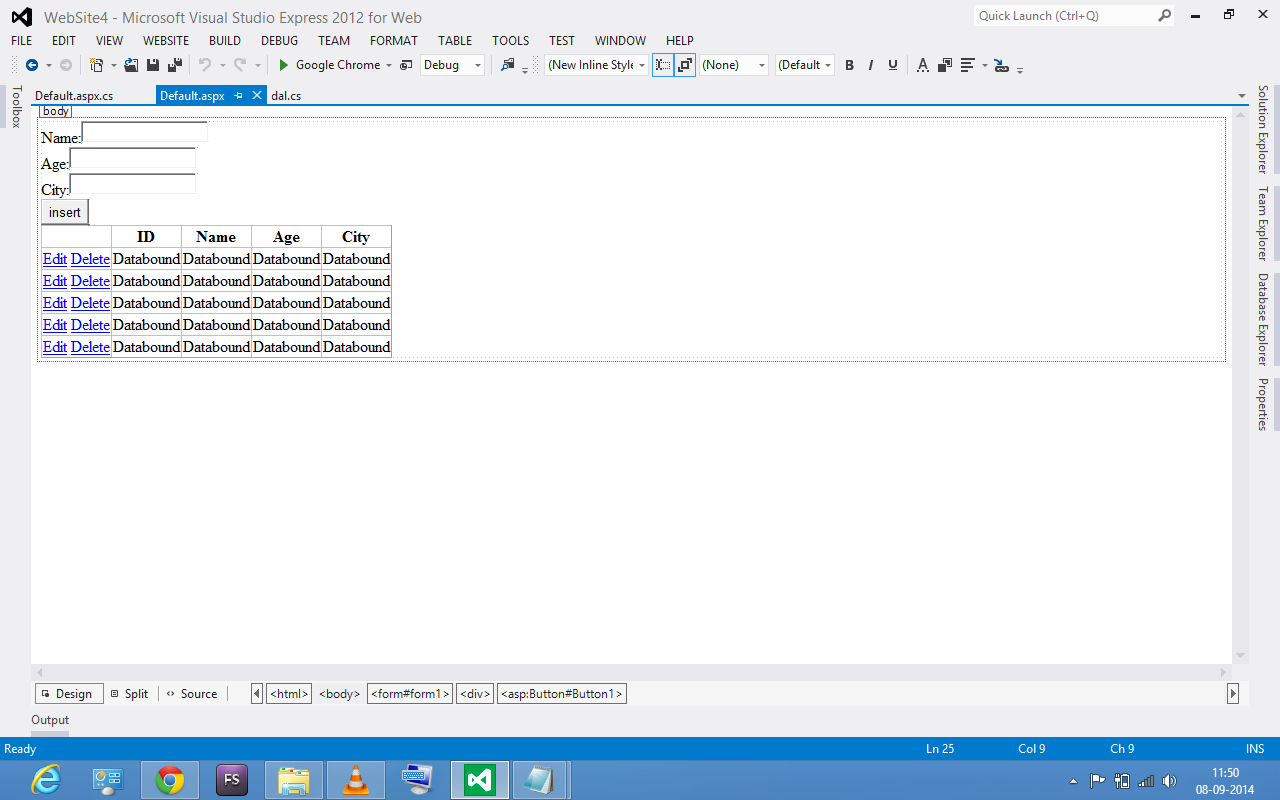
Step 5: In DAL File
Write that code You can difine your all logic here and also connection string
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using System.Data.SqlClient;
/// <summary>
/// Summary description for dal
/// </summary>
public class dal
{
SqlCommand cmd;
SqlConnection con;
SqlDataAdapter da;
DataSet ds;
public dal()
{
con = new SqlConnection(@"Data Source=TOPS-222\SQLEXPRESS;Initial Catalog=mytestdb;Integrated Security=True");
}
public DataSet disp()
{
cmd = new SqlCommand("disp",con);
cmd.CommandType = CommandType.StoredProcedure;
da = new SqlDataAdapter(cmd);
ds = new DataSet();
da.Fill(ds);
return ds;
}
public void insert(bal bl)
{
cmd = new SqlCommand("inserts", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("name",bl.name);
cmd.Parameters.AddWithValue("age", bl.age);
cmd.Parameters.AddWithValue("city", bl.city);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
public void update(bal bl)
{
cmd = new SqlCommand("updates", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("id",bl.id);
cmd.Parameters.AddWithValue("name", bl.name);
cmd.Parameters.AddWithValue("age", bl.age);
cmd.Parameters.AddWithValue("city", bl.city);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
public void delete(int a)
{
cmd = new SqlCommand("deletes", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("id", a);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
step 6:-In BAL File Create property and the create the DAL Object here.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
/// <summary>
/// Summary description for bal
/// </summary>
public class bal
{
public int id { get; set; }
public string name { get; set; }
public int age { get; set; }
public string city { get; set; }
dal dl = new dal();
public DataSet disp1()
{
return dl.disp();
}
public void insert1(bal bl)
{
dl.insert(bl);
}
public void update1(bal bl)
{
dl.update(bl);
}
public void delete1(int a)
{
dl.delete(a);
}
}
Step:7
.Default.aspx.cs Code File Write That Code..
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
bal bl = new bal();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
disp();
}
}
public void disp()
{
GridView1.DataSource = bl.disp1();
GridView1.DataBind();
}
protected void Button1_Click(object sender, EventArgs e)
{
bl.name = TextBox1.Text;
bl.age = int.Parse(TextBox2.Text);
bl.city = TextBox3.Text;
bl.insert1(bl);
disp();
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
int a = int.Parse(((Label)GridView1.Rows[e.RowIndex].FindControl("Label1")).Text);
bl.delete1(a);
disp();
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
disp();
}
protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex = -1;
disp();
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
bl.id = int.Parse(((Label)GridView1.Rows[e.RowIndex].FindControl("Label1")).Text);
bl.name = ((TextBox)GridView1.Rows[e.RowIndex].FindControl("TextBox2")).Text;
bl.age = int.Parse(((TextBox)GridView1.Rows[e.RowIndex].FindControl("TextBox3")).Text);
bl.city = ((TextBox)GridView1.Rows[e.RowIndex].FindControl("TextBox4")).Text;
bl.update1(bl);
GridView1.EditIndex = -1;
disp();
}
}
...
If you have any query then contact me
Its Realy Work........
ReplyDeletethanks bro
Delete